Writeup - One Byte (DUCTF 2023)
DUCTF 2023 - One Byte
Description
1 | Here's a one byte buffer overflow! |
Writeup
Here’s the source code (and binary):
1 |
|
The provided executable is a 32-bit dynamically-linked executable, with the following security measures:
1 | Arch: i386-32-little |
Finding the bug and determining how to get the flag is fairly easy. There’s a win()
function present which will give a shell when called. There is a 1 byte overflow in the main()
function. PIE is enabled, meaning we need an info leak to return to the win()
function, but the leak is given freely as “free junk” upon startup.
Using pwntools, the address of win()
was calculated from the init()
leak using the following code:
1 | # get WIN leak |
Stack Overflow
The hard part was figuring out how to use the one-byte overflow to control the $eip
register. Inspecting the program while running using GDB revealed that the one byte overflow overwrote the least significant byte of a stack address during a function ret
, meaning we have limited control over the stack pointer. Using that, we can shift what the stack looks like so when the $eip
register looks on the stack for the function return address, it points to an address that we specify.
The main drawback is that the stack addresses are randomized each time the program runs, meaning you don’t know where exactly your input on the stack is stored. To remediate it, you put the address on the stack as many times as possible, then overwrite the last byte with a random byte that ensures the values are aligned properly, and then you run the exploit many times until it finally meets the right requirements.
Solve Script
1 | from pwn import * |
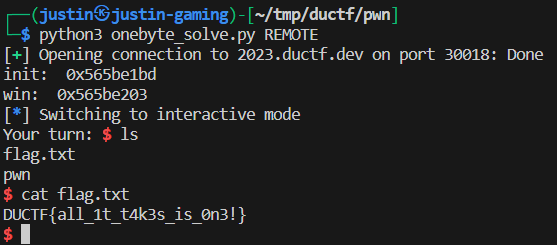
Flag: DUCTF{all_1t_t4k3s_is_0n3!}